Microsoft has recently introduced the first beta of its new stack for building great web sites – WebMatrix. One of the key components of WebMatrix is the ASP.Net Web Pages “Razor” Syntax (or simply: CSHTML) that lets you write C# code inside the HTML markup.
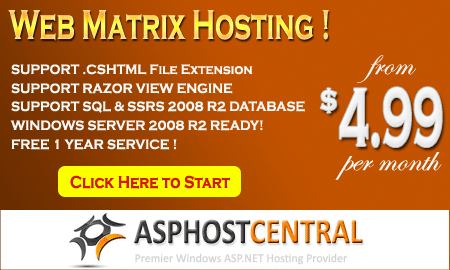
Helpers in WebMatrix
Among the new possibilities and simplicity that this approach brings to people who build web sites, there is also the notion of Helper. Helpers are a way to use a single line of code for a common task that originally had taken several lines of code to do. Such helpers can be:
- Sending mails
- Displaying a WebGrid for presenting tabular data
- Adding the Facebook button
- Querying the database
- Displaying a Twitter profile
- Embedding rich media (Silverlight, Flash, WMV)
In this post I will show how to create a custom helper to use in your ASP.Net Web Pages with Razor Syntax inside WebMatrix. For the demo I will build a TweetMeme helper that embeds the familiar TweetMeme button.
There are 2 ways of creating helpers to use in WebMatrix:
1. Create the helper as a Class Library.
2. Create it as a class and distribute the code.
Create CSHTML Helper for WebMatrix as a Class Library in Visual Studio 2010
1. Open Visual Studio 2010, and create a new Class Library that targets .Net Framework 4 called TweetMeme.Helpers.
2. Add a reference to System.Web.dll.
3. Rename the default file (Class1.cs) to a more meaningful name, such as TweetMeme.cs.
4. Rename the class name in that file to TweetMeme, and remove the namespace declaration. You better off without a namespace when using helpers.
In order to implement a custom helper, you should create a method that will render to required HTML for the helper. This method should be public and static method and should return IHtmlString as the following code:
public static IHtmlString Button(... parameters ... )
{
// Generate the HTML
string html = ...
return new HtmlString(html);
}
5. Implement the TweetMeme button method:
public static IHtmlString Button(string Url,
string Twitter = null,
bool Compact = false,
string UrlShortenerService = "bit.ly",
string UrlShortenerAPIKey = null)
{
// Generate the HTML
string html = GeneratedCode(Url, Twitter, Compact, UrlShortenerService, UrlShortenerAPIKey);
return new HtmlString(html);
}
6. Implement the GenerateCode method, that generates the HTML for TweetMeme:
private static string GeneratedCode(string url, string twitterName = null, bool isCompact = false, string UrlShortenerService = "bit.ly", string UrlShortenerAPIKey = null)
{
if (string.IsNullOrEmpty(url))
throw new ArgumentException("Url cannot be null or empty", url);
StringBuilder builder = new StringBuilder();
builder.Append("<script type=\"text/javascript\"> ");
if (isCompact)
builder.Append("tweetmeme_style = 'compact'; ");
builder.Append(string.Format("tweetmeme_url = '{0}'; ", url));
if (!String.IsNullOrEmpty(twitterName))
builder.Append(string.Format("tweetmeme_source = '{0}'; ", twitterName));
if (!String.IsNullOrEmpty(UrlShortenerService))
{
builder.Append(string.Format("tweetmeme_service = '{0}'; ", UrlShortenerService));
if (!String.IsNullOrEmpty(UrlShortenerAPIKey))
builder.Append(string.Format("tweetmeme_service_api = '{0}'; ", UrlShortenerAPIKey));
}
builder.Append("</script> ");
builder.Append("<script type=\"text/javascript\" src=\"http://tweetmeme.com/i/scripts/button.js\"></script>");
return builder.ToString();}
7. That’s it. The helper is ready to use.
Using a Custom Helper in WebMatrix
To use a custom helper in WebMatrix that is delivered as an assembly, you simply have to copy that .dll into a bin folder in your site.
1. In WebMatrix, add a bin folder to the root of your site.
2. Copy the output assembly of the helper (from the bin\debug\ folder of the class library you have just created) to the bin folder of the site.
3. In your code, simply call the Helper:
@TweetMeme.Button(url: Request.Url.AbsoluteUri,
twitter: "bursteg", // replace with your twitter name
compact: false)
Notice: If you now run your site locally, you with receive a TweetMeme button with a question mark in it. This is just because the url that it points to is a localhost address and not something that can be reached from the outside.
Create CSHTML Helper as a Class file inside WebMatrix
The second approach for creating helpers for WebMatrix is just adding a C# file with the helper class.
1. Inside WebMatrix, add a folder called App_Code to your web site.
2. Into the App_Code folder, add a new C# class to your web site.
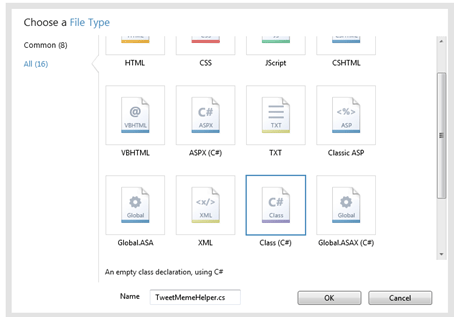
3. Create the helper in this file using the same code as above
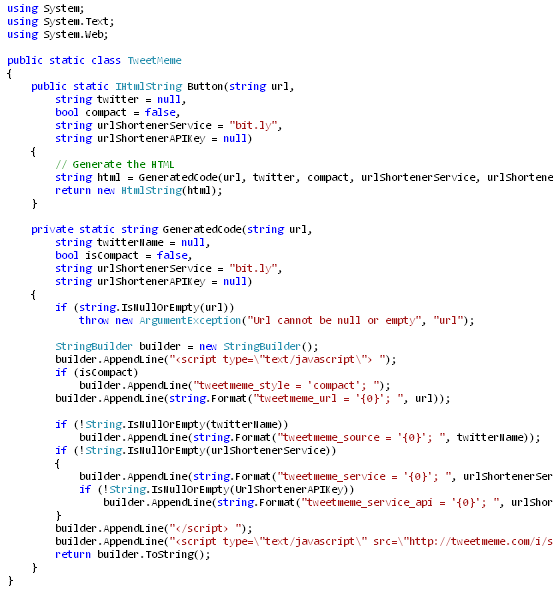
4. Use the helper in your code as shown above.
Conclusion
There are 2 approaches for creating Helpers for CSHTML. Creating helpers in class libraries are probably the better way of doing this in the eyes of professional developers, but my guess is that people who build web sites will prefer copying and pasting code samples for helpers to use in the project, and we will have a great community of helpers around the web.
Enjoy!