Introduction
Cookies are also known by many names, HTTP Cookie, Web Cookie, Browser Cookie, Session Cookie, etc. Cookies are one of several ways to store data about web site visitors during the time when web server and browser are not connected. Common use of cookies is to remember users between visits. Practically, cookie is a small text file sent by web server and saved by web browser on client machine.
Use of Cookies?
Cookies may be used for authentication, identification of a user session, user's preferences, shopping cart contents, or anything else that can be accomplished through storing text data. Cookies can also be used for travelling of data from one page to another.
Is Cookies Secured?
Well, this question has no specific answers in YES or NO. Cookies could be stolen by hackers to gain access to a victim's web account. Even cookies are not software and they cannot be programmed like normal executable applications. Cookies cannot carry viruses and cannot install malware on the host computer. However, they can be used by spyware to track user's browsing activities.
Using Cookies
Creating/Writing Cookies
There are many ways to create cookies, I am going to outline some of them below:
Way 1 (by using HttpCookies class)
//First Way
HttpCookie StudentCookies = new HttpCookie("StudentCookies");
StudentCookies.Value = TextBox1.Text;
StudentCookies.Expires = DateTime.Now.AddHours(1);
Response.Cookies.Add(StudentCookies);
Way 2 (by using Response directly)
//Second Way
Response.Cookies["StudentCookies"].Value = TextBox1.Text;
Response.Cookies["StudentCookies"].Expires = DateTime.Now.AddDays(1);
Way 3 (multiple values in same cookie)
//Writing Multiple values in single cookie
Response.Cookies["StudentCookies"]["RollNumber"] = TextBox1.Text;
Response.Cookies["StudentCookies"]["FirstName"] = "Abhimanyu";
Response.Cookies["StudentCookies"]["MiddleName"] = "Kumar";
Response.Cookies["StudentCookies"]["LastName"] = "Vatsa";
Response.Cookies["StudentCookies"]["TotalMarks"] = "499";
Response.Cookies["StudentCookies"].Expires = DateTime.Now.AddDays(1);
Reading/Getting Cookies
In the above code, I have used many ways to write or create cookies so I need to write here using all the above ways separately.
For Way 1
string roll = Request.Cookies["StudentCookies"].Value; //For First Way
For Way 2
string roll = Request.Cookies["StudentCookies"].Value; //For Second Way
For Way 3
//For Multiple values in single cookie
string roll;
roll = Request.Cookies["StudentCookies"]["RollNumber"];
roll = roll + " " + Request.Cookies["StudentCookies"]["FirstName"];
roll = roll + " " + Request.Cookies["StudentCookies"]["MiddleName"];
roll = roll + " " + Request.Cookies["StudentCookies"]["LastName"];
roll = roll + " " + Request.Cookies["StudentCookies"]["TotalMarks"];
Label1.Text = roll;
Deleting Cookies
In the above code, I have used many ways to create or read cookies. Now look at the code given below which will delete cookies.
if (Request.Cookies["StudentCookies"] != null)
{
Response.Cookies["StudentCookies"].Expires = DateTime.Now.AddDays(-1); Response.Redirect("Result.aspx"); //to refresh the page
}
Understanding HttpCookie Class It contains a collection of all cookie values.
We do not need to use any extra namespaces for HttpCookies class (we already have used this in Way 1 above), because this class is derived from System.Web namespaces. HttpCookies class lets us work with cookies without using Response and Request objects (we have already used this in Way 2 and Way 3 above).
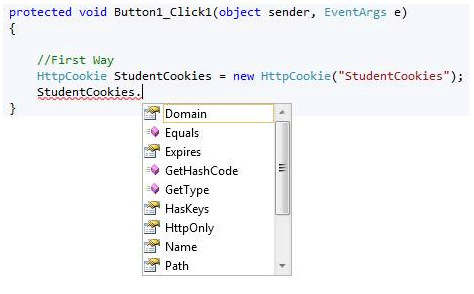
HttpCookie class has a list of some properties, let us outline them.
* Domain: It contains the domain of the cookie.
* Expires: It contains the expiration time of the cookie.
* HasKeys: It contains True if the cookie has subkeys.
* Name: It contains the name of the cookie.
* Path: It contains the virtual path to submit with the cookie.
* Secure: It contains True if the cookie is to be passed in a secure connection only.
* Value: It contains the value of the cookie.
* Values:
Limitations of Cookies
There are following limitations for cookies:
1. Size of cookies is limited to 4096 bytes.
2. Total 20 cookies can be used on a single website; if you exceed this browser will delete older cookies.
3. End user can stop accepting cookies by browsers, so it is recommended to check the users’ state and prompt the user to enable cookies.
Sometimes, the end user disables the cookies on browser and sometimes browser has no such feature to accept cookies. In such cases, you need to check the users’ browser at the home page of website and display the appropriate message or redirect on appropriate page having such message to enable it first. The following code will check whether the users’ browser supports cookies or not. It will also detect if it is disabled too.
protected void Page_Load(object sender, EventArgs e)
{
if (Request.Browser.Cookies)
{
//supports the cookies
}
else
{
//not supports the cookies
//redirect user on specific page
//for this or show messages
}
}
It is always recommended not to store sensitive information in cookies