With the introduction of ASP.NET MVC, the traditional ‘Control’ model of GUI component development went away. Instead it was replaced with the more Web 2.0 style JavaScript and jQuery plugin extensibility model. Nevertheless, adding incremental features to existing HTML components was always required and towards this end Microsoft introduced the HtmlHelper and helper extensions. Helper extensions are .NET extension methods that return a string. Typically, this string is formatted HTML content.
In our sample today we create an extension that renders a bar chart on HTML5 Canvas. It can be bound to a data source from the server side or be assigned data source from the client side.
Building a Html Helper Extension
Html Helper extensions are easy to build. Just follow the steps below to create our helper skeleton.
Step 1: We start with an MVC project and pick the Blank template.
Note we could pick a class library project template too and add package references as follows (via the Nuget package manager as follows)
a. PM> install-package Microsoft.AspNet.Mvc
b. Add reference to System.Web.dll from the ‘Add References’ dialog for the project
Step 2: Add a static class called ChartExtensions. Extension methods, by design, have to be defined in Static classes.
Step 3: Next add a static method Chart whose first parameters is this HtmlHelper and subsequent parameters are values required for rendering the chart.
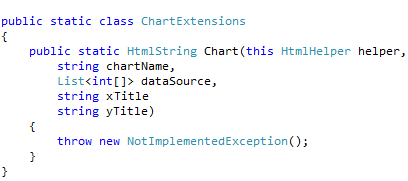
a. The dataSource is a list of integer arrays. For the example we have assumed a two dimensional array would be provided as a data source. We can make it more sophisticated by making it an array of objects and binding to different properties of the object.
b. xTitle: The text to be displayed on the x-axis.
c. yTitle: The text to be displayed on the y-axis.
Step 4: Next we setup two methods, one to convert the dataSource to JSON and the other to generate the required HTML.
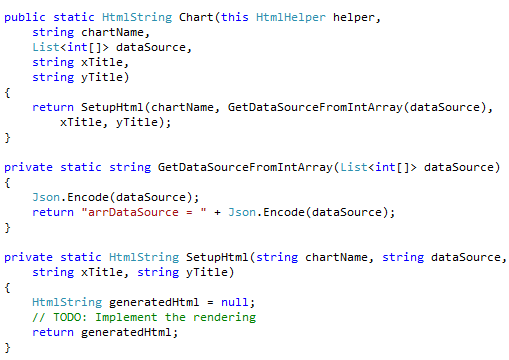
a. The GetDataSourceFromIntArray method uses the System.Web.Helpers’ Json static class to convert the input data source into a Json String. This string is assigned to a variable called arrDataSource and the whole string is returned.
b. The SetupHtml method implements the rendering logic
Step 5: Using TagBuilder to build the Html: ASP.NET provides us with the TagBuilder class that has helper methods to help create HTML elements easily. We use the TagBuilder to create our HTML layout as follows
<div>
<canvas …> … </canvas>
<script …> … </script>
<noscript> … </noscript>
</div>
The actual code is as follows
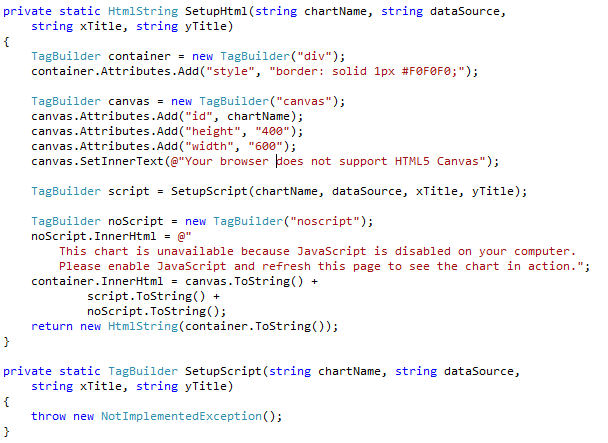
a. As seen above, the TagBuilder object has an Attributes collection to which we can add the HTML attributes we need. To set text between the opening tag and closing tag, we use the SetInnerText. However unlike an XmlTextWriter, we can’t nest tags. To nest tags we simply assign the string representation of the TagBuilder to the InnerHtml of the parent TagBuilder. So the ‘container’ Tag Builder has the <div> that encapsulates the Canvas and the Script tags.
b. We have created a 400x600 canvas area. The chartName parameter is used for the id of the canvas element.
c. For now we have an empty SetupScript method. This method will eventually build the JavaScript required to render the bar graph.
d. The <noscript> tag holds the message to display when JavaScript is not enabled on the browser.
e. Point to note is the HtmlString object that is returned. The HtmlString is a special object that indicates to the Razor view engine that it should not Html Encode this string any further. If we use string instead of HtmlString, the Razor engine automatically Html Encodes the string and we see the markup as text instead of rendered Html.
Step 6: The SetupScript method: The setup script method has the JavaScript that actually renders the chart on the canvas. The original code is borrowed (with permission) from this project. A demo for it is available here. We have to make the following changes so that it accepts any data source instead of the fixed data source assigned to it in the demo. We have also updated the code to use the strings passed in for x-axis and y-axis labels. Beyond this the animation and rendering code is pretty much the same.
a. The variable initialization change
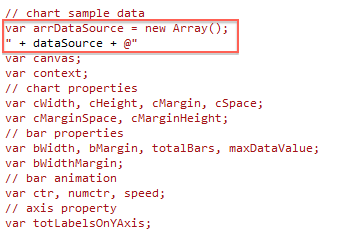
Earlier we had a hard-coded array of strings that was rendered on the canvas. Now we have the Json string that is created from the data source that was passed to the helper.
b. Changes to the barChart() method to set data source on the client side.
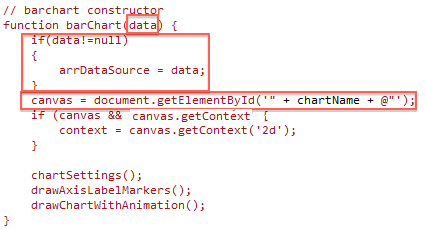
In the barChart method we earlier had no parameters and the elementId was hard-coded, now we use the value that’s passed into the helper.
We also pass a data parameter that is assigned to the data source of the graph.
c. Changes in drawMarkers() method to use the values passed into the helper for the x-axis and y-axis label
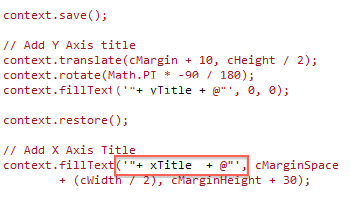
d. Changes to handle two-dimensional array of points instead of one-dimensional array of comma separated strings.
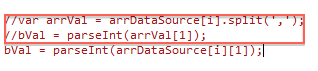
In the original code, the data source is an array of strings. Each element in the array has two comma separated values, represent the year (at index-0) and number of hits (at index-1). We have updated our data source to be a two-dimensional array and hence we don’t need to do a string split to get the value. We directly access the value using appropriate indexes. This change is at three places in the code. One of them is shown above.
With these changes in place our Chart, HtmlHelper is ready to be tested in an ASP.NET Web Application
Integrating the Custom Helper in a MVC Web Application
With our Helper all set, we add an MVC Web Application to our solution and call it DotNetCurry.HtmlHelpers.WebHarness. We use the Internet template that gives us a default Index.cshtml to put our Helper in. The following steps guide us through the integration effort
Step 1: Add Reference to the HtmlHelper project.
Step 2: Add a Model class in the Models folder.
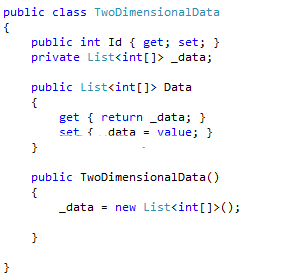
This is our view model that will hold the data to be rendered in the Graph.
Step 3: Update the HomeController to create and pass sample data model.
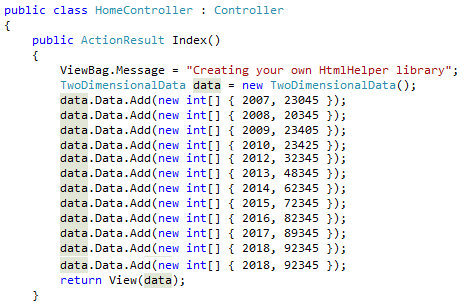
As seen above, we create an instance of our ViewModel class and add some test data into it. We pass this data on to the Index view, where the HtmlHelper will use it.
Step 4: Update the Index.cshtml markup to use the helper
The changes in Index.cshtml highlighted above, from top to bottom are as follows
a. Declare the model to be used in the page.
b. Add using to include the custom HtmlHelper
c. Added the HtmlForm inside which we use our Chart Helper extension. We give it an id=’sampleChart’, pass it the Model.Data and provide the labels for the x and y axes.
d. Finally in the jQuery document ready function, we initialize the chart by calling the barChart() method.
e. Our final effort looks as follows.
To change the graph change the data set coming from the controller.
That brings us to the end of this tutorial. We round off with some of the improvements possible.
Possible Improvements
1. Update the script to be able to bind to any Json object.
2. Use jQuery plugin syntax so that more than one helper can be created per page.
3. Allow more flexibility like passing parameters for bar colors, size of chart etc.
4. Allow multiple data sources to show comparison side by side.
5. Add more chart types like Pie etc.
Conclusion
Though they sound esoteric HtmlHelper extensions is a rather simple mechanism to inject rich functionality in a componentized way in ASP.NET MVC applications. We converted an existing HTML5 chart, originally created using plain HTML5 and JavaScript into a Chart HtmlHelper with very little code. For large projects, HtmlHelpers are a neat way to encapsulate small pieces of re-usable UI functionality
Introduction
MVC4 is really trying to break new ground in helping developers get to market more quickly and with a product that adheres to the emerging standards of today. A tough thing to ask of any technology, to be sure, but it's making some decent strides in the right direction.
Already the tutorials and blogs posts are popping up quicker than mushrooms on the midden heap. We highlight a few here that you definitely shouldn't miss which deal with key upcoming features...
ASP.NET MVC 4 Hosting
If you are looking for a QUALITY, AFFORDABLE host that supports ASP.NET MVC 4 Hosting, you can take a look at ASPHostCentral.com
ASP.NET MVC 4 Overview - Part 2
Jon Galloway goes into some significant detail here about some of the changes coming our way in the new version. He talks about the new default templates that come out of the box and the reasoning behind them.
He also talks about the new adaptive rendering taking advantage of CSS media queries and the usage of the viewport meta tag to help improve the mobile user experience. Overall a solid post that will stand the test of time. Check it out...
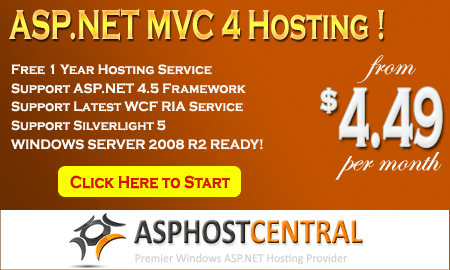
ASP.NET MVC 4 Bundling and Minification
Bundling and Minification of assets have to be two of the most tedious things to setup on a new web project. In this excellent post, David Hayden goes through exactly what you need to do to make the process as seamless as possible in MVC4.
Don't make the mistake of leaving this to the end of the project. You'll have deployment nightmares you didn't dream of and you'll be kicking yourself for not smoothing out the wrinkles. Do it early, and you'll thank yourself everyday for that small mercy...
Micro ORM Data Mapping with PetaPoco and ASP.NET MVC 4
Getting tired of working with the verbosity of Entity Framework classes and mapping? Well, you're not the only one, and in this quality post, Greg Arroyo takes us for a lap around the track using PetaPoco as your Micro ORM in MVC4.
Micro ORMs really are here to stay, and in some ways, more broadly applicable to web applications of today where everyone is trying to keep things more simple. If you haven't tried one yet, take this opportunity to get clued up on these fantastic tools.
ASP.NET MVC 4 Web API Routes and ApiController
Once again David Hayden masterfully takes us through a key piece of architecture that is going to be very important to anyone who will be working with MVC4. He details what seems to be a very slick piece of integration work between the new Web API framework and MVC4, and he throws in some OData queries for good measure.
It's short and sweet, and just enough to get you going, and whet your appetite for how to architect your solutions around your own APIs, allowing for more decoupled designs with less possible points of failure.
Getting started on your first Single Page Application
As with the introduction of any new method now encompassed in a technology, it's best to start with what comes straight from the horse's mouth. This Walkthrough put together by Brad Severtson gives you a great introduction, and leaves the mind boggling as to the possibilities.
If you've ever wanted to build your own Gmail and specifically wanted to do it with MVC, then go ahead, knock yourself out! (no pun intended)..
ASPHostCentral.com, the leader in ASP.NET and Windows Hosting Provider, proudly announces that we have supported the latest ASP.NET MVC 4.0 BETA Hosting.
To support Microsoft ASP.NET MVC 4.0 BETA Framework, we gladly inform you that we provide this beta account FREE of charge for a limited time (* terms and conditions apply).
New Features in ASP.NET MVC 4
This section describes features that have been introduced in the ASP.NET MVC 4:
- Enhancements to Default Project Templates
- Better Support for Mobile Project Template
- Enhancement in Display Modes
- Mobile Project Template support for VB.NET
- Dependency Injection Improvements
Terms and Conditions in Using this ASP.NET MVC 4.0 BETA Account
The followings are the features you will get under this FREE ASP.NET MVC 4.0 BETA Account:
- ASP.NET MVC 4.0 Beta Framework
- 1 Website/Domain
- 100 MB disk space
- 100 MB bandwidth
- 50 MB SQL 2008 space
- 24/7 FTP access
- Windows Server 2008 Platform
If you want to participate in this BETA program, there are several rules you need to understand:
- As this is a beta version, not all the features are available. They may be some issues on this beta framework, which will be fixed upon the full release of ASP.NET MVC 4.0 Framework
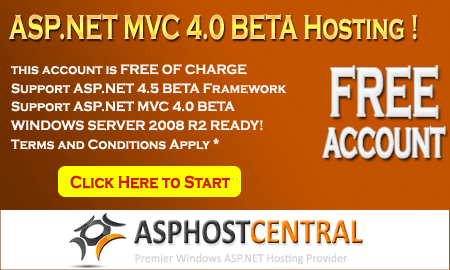
- ASPHostCentral.com does not guarantee the uptime of the sandbox solution. Additionally, we do not keep/store any backup of your files/accounts
- ASPHostCentral.com does not guarantee rapid response to any inquiries raised by a user
- This free account is only meant for testing. Users should not use it to store a production, personal, e-commerce or any blog-related site
- This free account is used to host any ASP.NET MVC 4.0 beta website only. Any questions that are not related to ASP.NET MVC 4.0 BETA will not be responded. A user shall not host any non-ASP.NET MVC 4.0 site on this free account either
- ASPHostCentral.com reserves full rights to terminate this beta program at any time. We will provide a notification on our Help Desk System prior to the termination of this program
- ASPHostCentral.com reserves full rights to terminate a user account, in which we suspect that there is an abuse to our system
- Once this beta program is terminated, your account will be completely wiped/remove from our system.
- This offer expires on 31st May 2012
If you wish to participate in this FREE ASP.NET MVC 4.0 BETA Program, you must register via https://secure.asphostcentral.com/BetaOrder.aspx