Logging exceptions is important for controlling your application when they are deployed. You can opt for using one of the available libraries on the market or your own way of storing this information. Both sides have their own pros and cons. Using a third-party code lets you implement the task in less time.
Writing your own code is probably a win situation if you do not want to include reference to gigantic libraries in order to only use a small part of their features. Handling errors the right way is crucial from the security point of view: the less your attacker sees, the more secure your application. In this article, you will learn how to protect your error from others and, at the same time, log it for tracking purposes
Error logging with Enterprise Library and log4Net
If you decide to use custom libraries to handle logs, you will probably choose between Microsoft Enterprise Library and Apache Foundation log4net. Microsoft Enterprise Library, at the time of writing, is available in version 4.1 at http://msdn.microsoft.com/en-us/library/cc467894.aspx. This library is free and contains a lot of functionalities; logging is only a small part of it. It is diffused among enterprise applications because even though it is not part of the .NET Framework BCL, developers tend to trust external class libraries coming from Microsoft.log4net is a project from Apache Software Foundation and it is available under the Apache License at http://logging.apache.org/log4net/index.html.
Both libraries provide great flexibility; you can log information (and errors) to a file, a database, a message queue, or the event log or just generate an e-mail.
If you are trying to choose one over the other, you have to consider these points:
- Enterprise Library has a GUI tool to configure its Logging Application Block
- log4net supports hierarchical log maintenance
The choice is based mainly on the features you need to address because, from the performance point of view, they are very similar.
Enterprise Library is often considered because of its capabilities so, if you are already using it in your project (for example, because of the Cache Application Block), you may find it very similar, and using it is the right move because you already have a dependency on the main library
On the other hand, log4net is preferred by developers searching.
only for a simple and very complete library to perform this task and nothing more
If you prefer to write code, however, and your logging needs are only related to exceptions, you'll probably find easier to just handle and store this information with your custom code
Intercepting and handling errors with a custom module
Exposing errors to end users is not a good idea from both the usability and the security point of view. Error handling implemented the right way will help the administrators to inspect the complete error and provide a courtesy page to the users.
Problem
We want to avoid full error disclosure to normal users and display the full error to the administrators. This will preserve security and help the administrators to inspect errors without accessing the error logging tool while they’re running the page causing the error. We want to provide also an entry point to add more powerful exception logging capabilities in the future
Solution
ASP.NET gives you control over errors, letting you choose from among three options:
- Always show the errors
- Never show the errors.
- Show the error only when the request is coming from the same machine running the application
The following code comes from a typical web.config and lists the options:

These settings are flexible enough to cover your needs while developing the application; the reality is that, when you put your application in production, you will probably not make requests from the same machine running the page and you need to be the only one accessing error details
It is very important to not show sensitive information to users: errors are considered very dangerous. HttpApplication has a useful Error event, used to intercept exceptions not blocked at a higher level, such as in a try/catch block. This event can be handled to combine authorization and authentication from ASP.NET so you can show the error only to a specific group of people, thanks to Roles API available on ASP.NET
The code is really simple: you just have to handle the event, verify user permissions given the current roles, and then show a personalized error page or just let ASP.NET do the magic, using the values specified in web.config
We need to configure web.config to register our module as in listing 1
Listing 1: The custom authorization module to modify the response flow
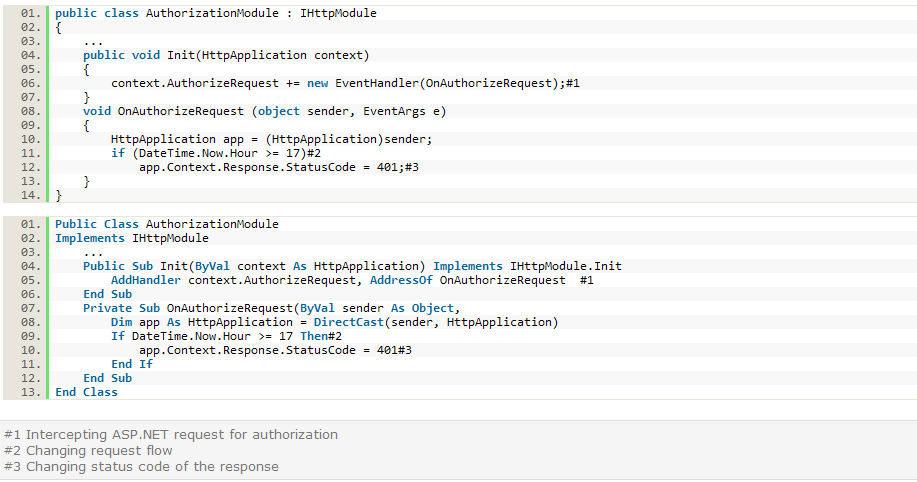
When an error occurs, the exception is handled by our event handler, and we will display an error message similar to the one in figure 1
Figure 1: Using our custom error system we can add further information to the error page or simply decide to show the error to given clients.
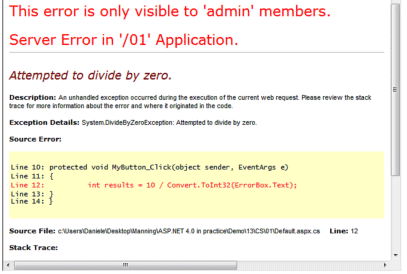
To implement such a personalized view, we need to write a custom HttpModule like the one in listing 2
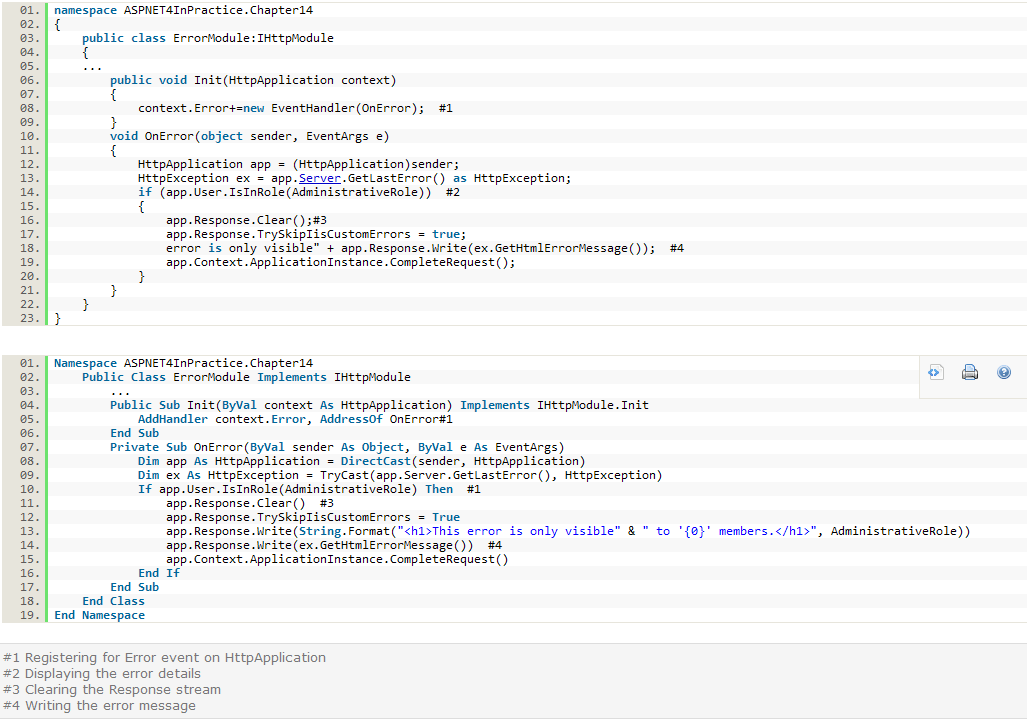
This code can be easily adapted to integrate further logging instrumentations, like form variables or application status. To register the module, you have to place this configuration in your web.config:
Error event handler is the right place to add your code. You can use MailMessage class from System.Net.Mail to compose a notification email and send it to your address. If you want to use something that’s readily available, take a look at Health Monitoring in the MSDN documentation.
It is important to remark that TrySkipIisCustomErrors property from HttpResponse class is used to modify the default behavior of IIS 7.x when dealing with custom errors. By default, in fact, IIS 7 will bypass the local error handling and, instead, use the configuration applied in the system.webServer section. By setting this property, you can control IIS 7.x behavior too; the behavior of IIS 6.0 is not affected by this change
Discussion
HttpModules enable global event handling and are very useful in such a situation. This approach is very simple, centralized, and open to further improvements. It is also showing you how easy it is to tweak ASP.NET behavior and to avoid security concerns: the less an attacker sees the better it is for your application security. Error logging can be done with many different approaches. What we showed in these examples is a starting point. To meet your more complex needs, you can use the libraries we mentioned
Summary
Remember that ASP.NET is built with flexibility. This characteristic reflects how many incredible things you can do using extreme techniques. ASP.NET offers the right entry points to add your own custom mechanisms to implement simple things like logging errors. ASP.NET is so powerful that you can literally do anything you may need; you just have to write code and unleash your imagination!
