The Good News - In ASP.NET 4.5, we can adopt an approach using which the Model can be directly bound with the DataBound controls and CRUD and pagination operations can be implemented very effectively. It incorporates concepts from the ObjectDataSource control and from model binding in ASP.NET MVC. We will see this shortly. ASP.NET 4.5 is based upon .NET 4.5 and it gets installed once you install Visual Studio 2011 preview.
Note: If you want to install Visual Studio 2011 preview, you can also use the Windows 8 Developer preview.
In this article I will be explaining a new ASP.NET 4.5 exciting feature called ‘Model Binding with Web Forms’. Up to previous versions, webforms for data-binding used to make use of the ‘Eval’ method. During runtime, calls to Eval makes use of reflection against the currently bound data object and reads value of the member with the given name in Eval method. (Read Why Eval is Evil). Once this value is read the result is displayed in HTML. Although this is easiest way of data-binding, it has limitations like checking the binding name during compilation time etc.
Update: Also check out the second part of this article ASP.NET 4.5: Filtering using Model Binding in ASP.NET Web Forms
In ASP.NET 4.5 the Model Binding has improved. We will be going through the complete model binding feature using the following steps:
- Model binding with Web Forms.
- Value Providers.
- Filtering.
- Performing Update Operations.
For this article I am using Sql Server 2008 R2 and a ‘Company’ database, with following Tables:
Department - DeptNo (int) Primary Key, Dname (varchar(50)),Location (varchar(50)).
Employee - EmpNo (int) Primary Key, EmpName (varchar(50)),Salary (int), DeptNo(int) Forwign Key.
Let’s get started.
Step 1: Open Visual Studio 2011 Developer Preview and create a new Web Application, make sure that the Framework version you select is .NET 4.5. Call this application ‘ASPNET45_ModelBinding’.
Step 2: In this project, add new folders and name them as Model and Department. In the Department folder, add two Web Forms (with master page). Name them as ‘Departments.aspx’ and ‘DepartmentDetails.aspx’.
Step 3: In the Model folder, add a new ADO.NET entity data model and name it as ‘CompanyEDMX.edmx’. In the Wizard, select Company Database and select Department and Employee table. After the completion of wizard, the below result will be displayed:
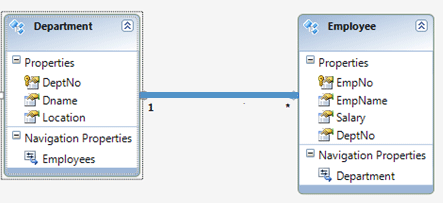
Step 4: Open the Departments.aspx in the ‘Source’ view and add the Repeater control in it with the Department model bound to it as below.
The above code shows some modified databound features for DataBound controls in ASP.NET 4.5. The Department Model is assigned to the ‘ModelType’ property of the repeater. This property is available to all DataBound controls. This allows us to define the type of data that is bound to the control and also allows to bind properties of the Model inside the control. The above code defines ‘ItemTemplate’ inside the repeater control which refers to the ‘DepartmentDetails.aspx’ by passing DeptNo value using QueryString to it.
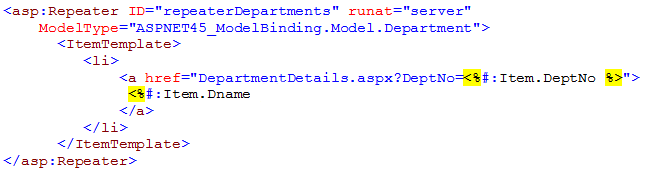
Step 5: Go to the Departments.aspx.cs code behind, and write the following code:
The above code sets the datasource property for the repeater control.
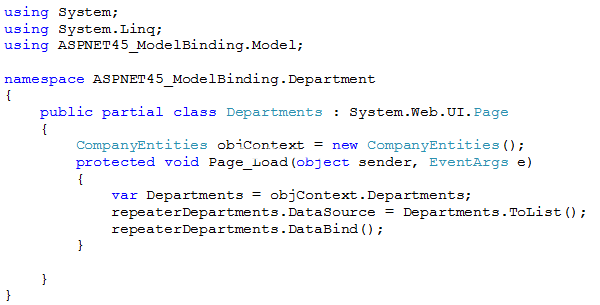
Step 6: View the Departments.aspx inside the browser and the following result will be displayed:
In your OS, observe the lower right corner of the System Tray. Instead of the ASP.NET Development server, ASP.NET 4.5 uses IIS Express as shown below:
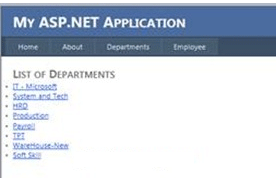
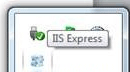
Step 7: In Step 4, we added the repeater control which has the ItemsTemplate and contains an <a href=””> to navigate to DepartmentDetails.aspx using a query string. This page is designed for displaying details of a particular Department. Open DepartmentDetails.aspx in the ‘Source’ view and add a DetailsView web UI databound control inside it. As explained Step 4, we need to assign the ModelType property of this control to ‘Department’ model.
All those who have used controls like DetailsView or FormView knows that these control are used for performing DML operations. Now to perform DML operations in earlier versions of ASP.NET i.e. from 2.0 to 4.0 we used to make use of ObjectDataProvider and this provider was usually configured using Get,Insert,Update and Delete methods form the source object. However the ASP.NET 4.5 DataBound controls e.g. GridView, FormView, DetailsView etc, exposes the following properties:
- SelectMethod: Used to make call to a method which returns IEnumarable.
- InsertMethod: Used to make call to a method which performs Insert operation.
- UpdateMethod: Used to make call to a method which performs Update operation.
- DeleteMethod: Used to make call to a method which performs Delete operation.
Configure the DepartmentDetails.aspx as shown below:

Step 8: Open the DepartmentDetails.aspx.cs and add the following code in it:
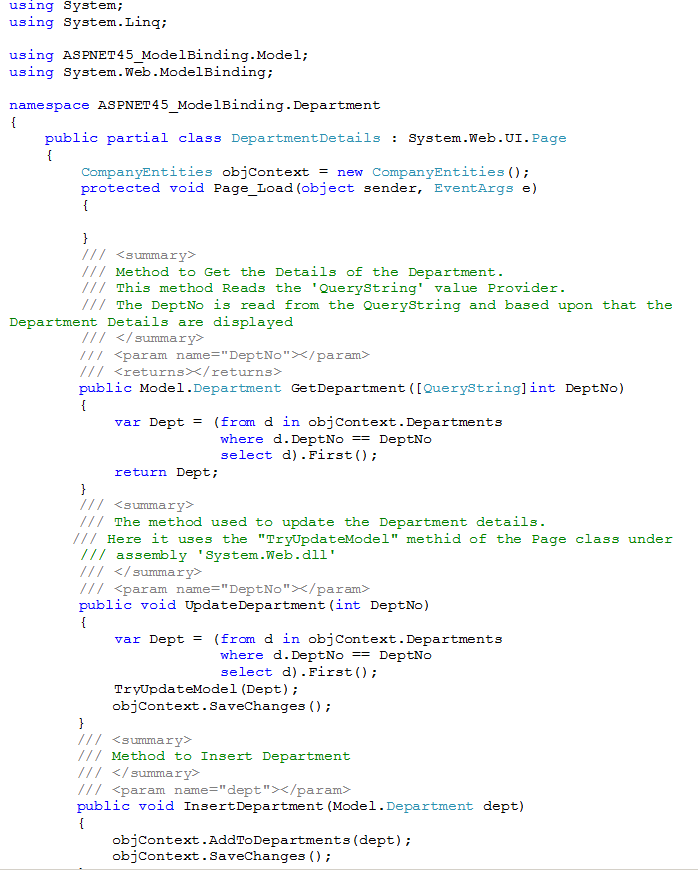
Now carefully have a look at the above methods. None of these methods make use of any of the UI controls in the user interface. All these methods strictly work on Model objects and this feature drastically reduces additional coding. One more important fact is, if you observe the ‘GetDepartment()’ method, it has defined the ‘DeptNo’ input parameter with the QueryString Value provider. This automatically reads the DeptNo in the QueryString and displays the Department details inside the DetailsView.
Note: In previous versions of ASP.NET we could have done this using Request.QueryString[“DeptNo”]
Step 9: Now open Site.Master and add the following menu item:
<asp:MenuItem NavigateUrl="~/Department/Departments.aspx" Text="Departments"/>
Step 10: Make Default.aspx as a startup page and run the application. You will see the Default.aspx with Department and Employee menu. Once you click on ‘Department’ menu, Departments.aspx will be displayed. Now click on any Department and you will be transferred to ‘DepartmentDetails.aspx’ as below:
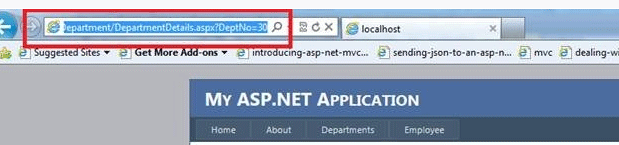
The QueryString has the DeptNo and based upon the value of the DeptNo, the DetailsView will display the Department details. Here you can now test the Update and New (insert) functionality.
Check out the second part of this article ASP.NET 4.5: Filtering using Model Binding in ASP.NET Web Forms
Conclusion: The Model binding feature provides facility to the developers to develop Webforms which can be independent from the Model